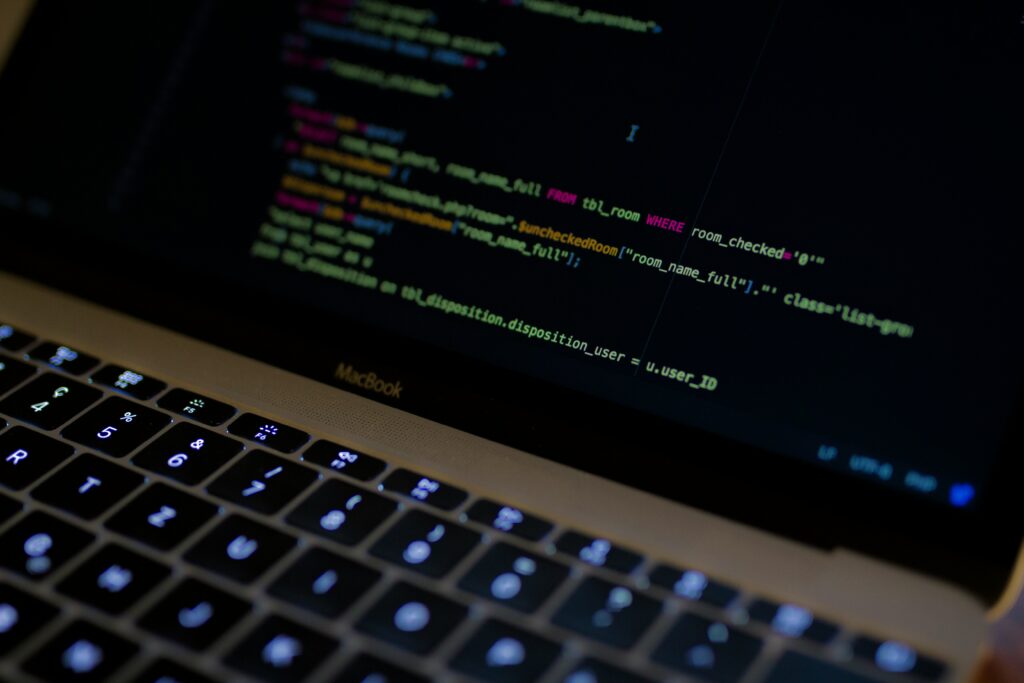
Hooks in React is one of the most significant innovations in the library ecosystem. They appeared in React 16.8 and radically changed the way we manage the state and side effects of the functional components.
In this article from Celadonsoft, we are going to give you a React Hooks Cheat Sheet, that will help you during the development process.
Why Did the Hooks Appear?
Before they came, developers had to use class components for state (setState) and life cycle (componentDidMount, componentDidUpdate). This led to a number of problems:
- Complexity of syntax and logic – code inside life cycle methods often became confusing, especially when working with asynchronous data.
- Problems with reusing logic – overused logic had to be output in HOC (Higher Order Components) or Render Props, which complicated the code base.
- Complexity of working with classes – the context of this in class components typically led to bugs and required explicit binding (bind).
How Do the Hooks Solve These Problems?
Hooks allow:
- Use the state inside functional components, eliminating the need to write classes.
- Divide business logic into more compact and re-used hooks, which makes the code cleaner and easier to accompany.
- Get rid of the context, this – now working with state and effects is intuitively understandable.
Benefits of Using Hooks
- Clean code – functional components become more concise and easier to understand.
- Flexibility – can be divided the logic of the state and side effects by the meaning blocks, rather than distributing it between life cycle methods.
- Better control over the reindeer – with hooks (useMemo, useCallback) you can optimize performance.
- Ease of testing – the functional approach makes it easier to test components.
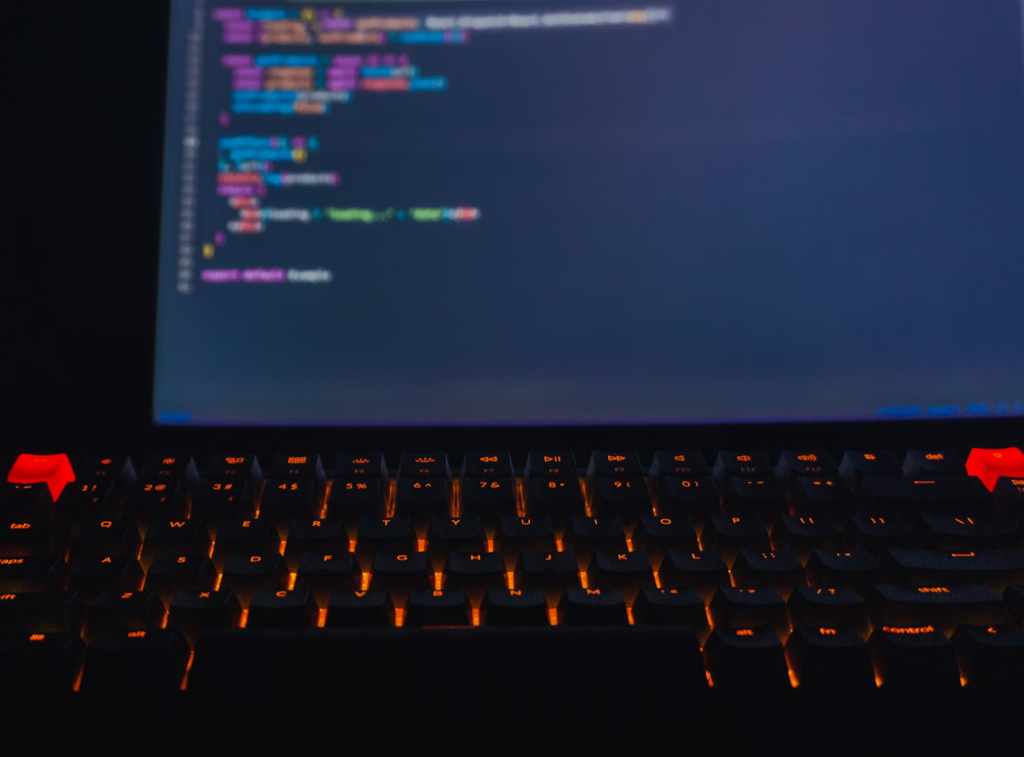
Basic Rules for Using Hooks
The main rule from the MVP development company Celadonsoft: “The correct use of hooks is key to their effectiveness and prevention of possible errors.” To avoid problems and use hooks as effectively as possible, it is worth following a few basic rules.
Hooks Are Only Called at the Top Level
This is one of the most important rules. Hooks from our React Hooks Cheat Sheet cannot be called within conditions, cycles or embedded functions. All hooks must be called at the top of a functional component or other hook. This allows React to ensure the correct order of hooks are called in each component’s render.
Hooks Can Be Triggered Only in Functional Components or Other Hooks
It is critical to remember that hooks can only be used inside functional components or other custom hooks. They do not work in classes, and cannot be called in normal JavaScript functions.
Follow the Dependencies in useEffect and Other Hooks
When using the useEffect hook, it is essential to correctly specify the dependencies in order to avoid unnecessary renderings and side effects. If the dependencies are not specified or are not correctly specified, this can lead to infinite renders or incorrect execution logic.
Using ESLint to Check Hooks
To avoid common hook errors, we recommend using a special ESLint plugin – eslint-plugin-react-hooks. This plugin automatically checks for correct use of hooks and reminds you of rules to prevent them from being broken.
The Right Management of the State
When using useState, it is indispensable to remember that state changes can be asynchronous. Keep an eye on how the data is updated and what data you use to update it.
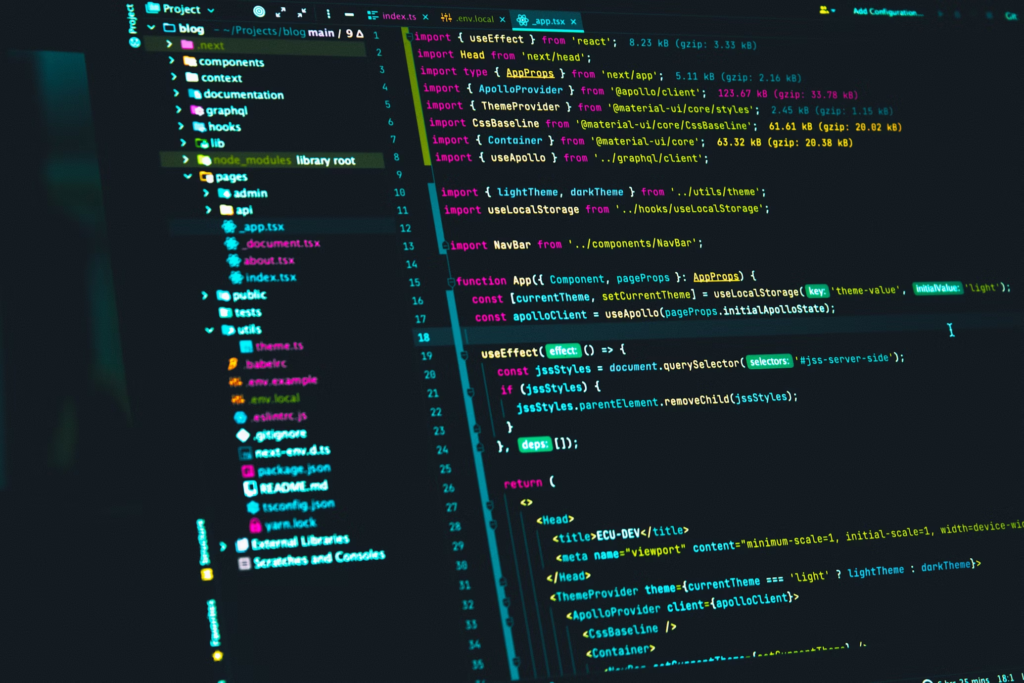
Review of the Main Hooks
In this section we will look at the three most popular hooks from the Celadonsoft React Hooks Cheat Sheet that are used to work with state, side effects and context in React.
useState – Component State Management
useState is a basic hook that allows you to add a local state to functional components. It takes the initial value of the state and returns a pair: the current value and the function to update it.
This hook is extremely useful for simple state management scenarios such as tracking values in forms, switching UI states, etc. It is also useful for the following.
useEffect – Side Effects Processing
useEffect allows you to manage side effects in components: data loading, DOM change, subscriptions etc. Hook is called after component renderer and can be configured to run when certain dependencies are changed.
useEffect is ideal for handling asynchronous queries, timers, or cleaning resources such as unsubscribing or unmounting component requests.
useContext – Access Context Without Append
useContext simplifies working with React contexts by eliminating the need to pass data through props in each component. This is especially useful for global states, such as theme layouts, authentication, and interface language.
Using useContext allows you to avoid “rolling” data across several component levels and makes the code more readable.
Additional Hooks for Advanced Use
Once you have mastered the basic hooks from Celadonsoft React Hooks Cheat Sheet , it is time to move on to more complex and powerful tools. These hooks will help you optimize your performance and work with more complex cases.
useReducer – Managing Wealth Through Resources
If the state of a component becomes too complex for useState, it is better to use useReducer. This hook is similar to the work with Redux, where we describe a state change ruler that is ideal for more complex logic.
useReducer is ideal if your component manages multiple state values or if you need to organize complex actions.
useCallback and useMemo – Performance Optimization
These hooks allow you to avoid unnecessary components re-render, which can be useful in large applications. useCallback memorizes functions, and useMemo computations so that they are not recalculated in each render.
useMemo can be useful for the memorization of complex calculations that depend on changing data but do not have to be recalculated in every render.
Rare but Useful Hooks
There are a few hooks in our React Hooks Cheat Sheet that are less common in everyday practice, but they greatly extend the capabilities of React, especially in specific cases.
useDeferredValue – Delayed Value Processing
This hook helps to delay value updates so that the interface remains responsive even if the data is processed for a long time. This hook is useful when implementing complex filters or search queries where you need to delay actions without blocking the interface.
useTransition – Update Priority Management
useTransition allows you to manage the priority of the API rendering, which is useful for creating smoother user interfaces. This helps to split the interface into parts with different priorities.
useTransition helps to improve the UX when you need to update part of the UI without blocking the rest of the interface.
useId – Unique Identifiers
useId helps to generate unique IDs for components, which is important for accessibility and testing. Unlike Math.random(), useId generates identifiers that are consistently unique within the framework of rendering. This hook is useful for creating accessible forms and ensuring the uniqueness of identifiers in components.
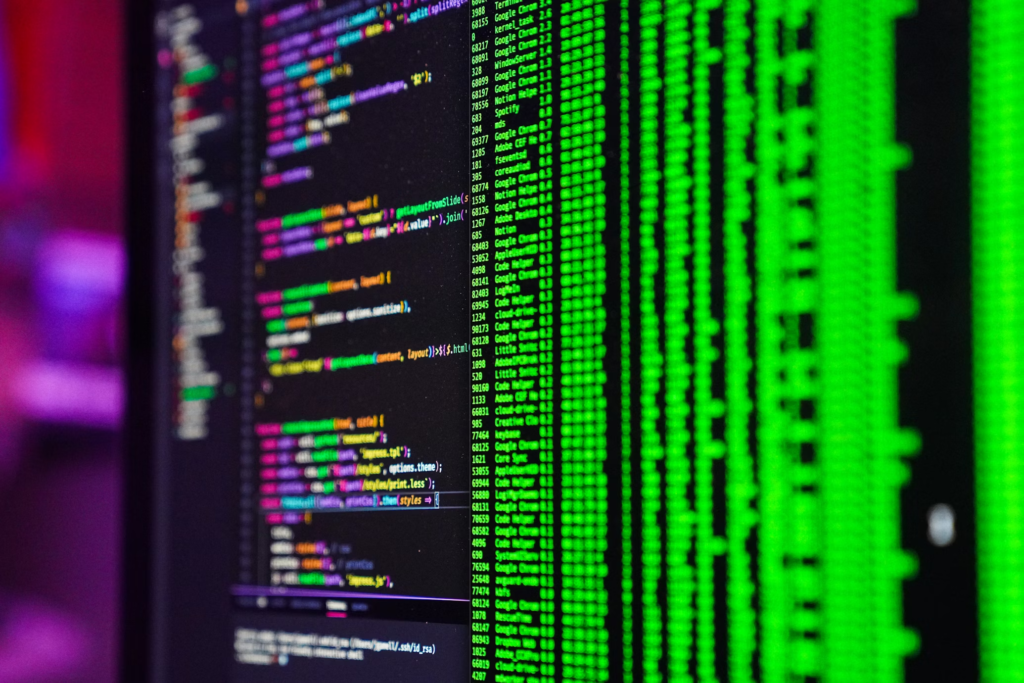
Common Mistakes and Pitfalls When Using React Hooks
Using hooks in React can make development much easier, but it also has a number of pitfalls that are indispensable to keep in mind to avoid performance issues or bugs. Here are some common mistakes and ways to avoid them:
- Hook violation: One of the main mistakes is to violate the fundamental rules of hooks. Hooks should only be called at the top of a component, and cannot be called within conditions or cycles. Breaking these rules can lead to unpredictable results and even app failures.
- Dependency errors in useEffect: When using the useEffect hook, it is significant to correctly indicate the dependencies. If you forget to specify the dependencies or if they are not correctly specified, it will lead to unnecessary constraints or, conversely, to their absence when they are required. Using ESLint plugin with hook rules will help avoid similar mistakes.
- Endless render cycles: Infinite rendering cycles can occur due to improper operation with state hooks, for example when changing state inside useEffect without proper dependencies. This will lead to a component regenerator, which in turn will again cause the effect to go on and on.
- Errors when working with refs (useRef): Using useRef to save changing data without a component re-maker may not be obvious to beginners. It is important to remember that useRef keeps the reference to the object, but changing this object does not cause a component to be overridden, which can be useful in some cases, but in others, on the contrary, lead to errors.
Conclusion: Why should we delve into React hooks?
React hooks greatly simplify development and make the code cleaner and more supported. They eliminate the need for classes and provide a more direct way of working with state and side effects.
Advantages of using hooks:
- Cleaner and more compact code: hooks allow avoiding unnecessary nesting and the pattern code.
- Better logic repeatability: you can easily create and reuse custom hooks.
- Ease of testing: hooks can be tested in isolation, which makes unit testing easier.
Recommendations for further study: If you are already familiar with basic hooks, we strongly recommend that you study more complex patterns and examples of their use. For example, custom hooks for managing the state or processing data, and hook integration with TypeScript for typing data. You can also start using hooks to work with asynchronous queries with useEffect or for more complex business logic.
Remember best practices and follow the rules to avoid common mistakes. Tools like ESLint and React DevTools will help you to debug and improve your application.